项目作者: SAshwinAchu10
项目描述 :
Event, Logs Tracer
高级语言: TypeScript
项目地址: git://github.com/SAshwinAchu10/data-tracer.git
Data Tracer
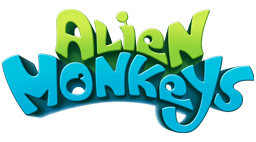
Installation
$ npm i data-tracer
Import
import { Audit, DataTracer } from 'data-tracer';
Instantiate Tracer
Mongoose
Audit.addTracer('mongoose', { connectionString: 'mongodb://localhost:27017/db', appName: 'Apple-Rest' });
Mongo Aggregation Query
import { Audit } from 'data-tracer';
app.get('/audits', async (req: any, res: any) => {
let query: any = req.query;
if (query['page'] == undefined) query['page'] = 1;
if (query['limit'] == undefined) query['limit'] = 10;
let q: any =
query['q'] == undefined
? [{}]
: [
{ when: { $regex: `${query['q']}`, $options: 'i' } },
{ what: { $regex: `${query['q']}`, $options: 'i' } },
{ type: { $regex: `${query['q']}`, $options: 'i' } },
{ where: { $regex: `${query['q']}`, $options: 'i' } },
{ why: { $regex: `${query['q']}`, $options: 'i' } },
];
return Audit.aggregate([
{
$facet: {
audits: [
{
$match: {
type: { $in: JSON.parse(query['type']) },
createdAt: {
$gte: new Date(query['startDate']),
$lte: new Date(query['endDate']),
},
$or: JSON.parse(JSON.stringify(q)),
},
},
{ $limit: parseInt(query['limit']) },
{ $skip: (query['page'] - 1) * query['limit'] },
],
apps: [
{
$group: {
_id: null,
apps: { $addToSet: "$appName" }
}
}, { "$unset": ["_id"] }
]
},
},
]).then((result: any) => res.json({ data: result }));
});
MySQL
Audit.addTracer('mysql', { host: 'localhost', user: 'root', 'password': 'root', database: 'myDB' });
Instantiate Mail Alerts (Optional)
DataTracer.configureAlert({
'apiKey': 'abcdefghijklmnopqrstuvwxyz',
'provider': 'sendgrid',
'subject': `Error from ${APP_NAME}`,
'from': 'achu10@live.in',
'mailTo': 'achu10@live.in',
'type': ['SEVERE'] // Severity types to trigger email
});
Sample usage
Definition
Audit.logEvent(
type,
what,
subject,
status,
who,
where,
why,
is,
meta
);
Example
Audit.logEvent(
'INFO',
'Ping',
'api was triggered',
'Successful',
undefined,
'app.js',
'to Check Server status',
'Event',
{
'key1': value,
'key2': [
{
'key21': 'value21'
}
]
}
)
Examples
Screenshot

Roadmap [WIP]
- Local file logging
- Dashboard UI
- QL for filtering Audit Events, Logs
- Plugins like console, authorization etc.
- ELK Integration
- Monitoring
- Tracing
- etc…